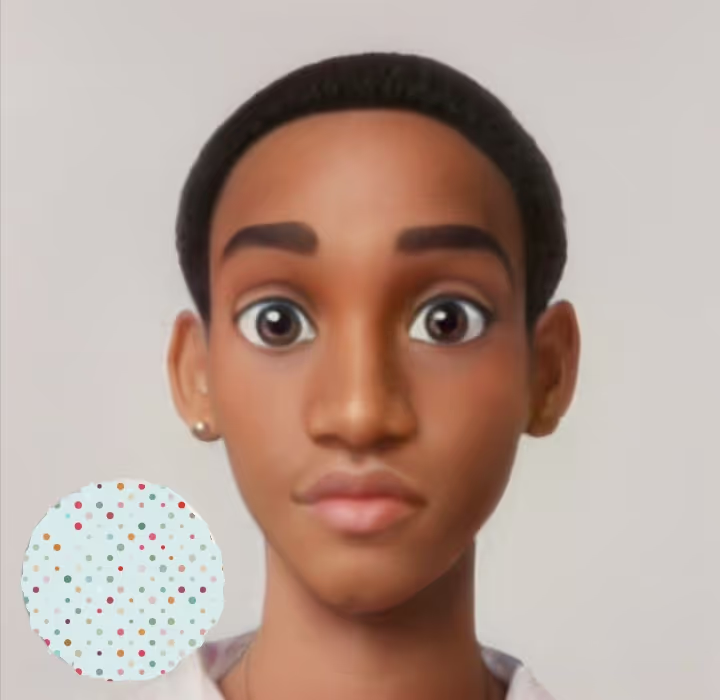
Golang String Manipulation
Strings in Go
In Go, strings are a fundamental data type. They are immutable, meaning they cannot be changed once created. Strings are made up of a sequence of bytes and are UTF-8 encoded. This means that each character in a string is represented by one or more bytes. For example, the character “é” is represented by two bytes: 0xC3 and 0xA9. The Go language specification defines a string as a sequence of bytes, not necessarily characters. This means that a string can contain any sequence of bytes, including null bytes, and it is up to the user to determine how those bytes should be interpreted.
Creating Strings in Go
To create a string in Go, you can use either double quotes or backticks. Double quotes are used to create a string literal, while backticks are used to create a raw string literal. A string literal is a sequence of characters surrounded by double quotes or backticks. A raw string literal is a string literal that is interpreted literally, without any escape sequences.
...
s1 := "Hello, World!"
s2 := `Hello, World!`
fmt.Println(s1 == s2) // true
How to Create multiline strings in Golang
To create a multiline string in Go, you can use backticks.You could also use the +
operator to concatenate multiple strings, but this is not recommended because it is less efficient.
...
query := `SELECT * FROM users
WHERE id = 1 AND name = "John"`
String Manipulation in Go
Go provides a powerful set of tools for manipulating strings. Whether you’re looking to do basic slicing and dicing, more complex pattern matching, or something else entirely, Go has you covered.
How to Get the Length of a String in Go
To get the length of a string in Go, you can use the len
function. This is possible because Go strings are made up of a sequence of bytes. The len
function returns the number of bytes in a string.
...
s := "Hello, World!"
fmt.Println(len(s)) // 13
How to trim whitespace in Go
To trim whitespace from a string in Go, you can use the strings.TrimSpace
function. This function takes a string as an argument and returns a string with all leading and trailing whitespace removed.
...
s := " Hello, World! "
fmt.Println(len(s)) // 15
s1 := strings.TrimSpace(s)
fmt.Println(s1) // Hello, World!
fmt.Println(len(s1)) // 13
To trim whitespace from the left side of a string, you can use the strings.TrimLeft
function. This function takes two arguments: the string to trim and the set of characters to trim. It returns a string with all leading characters in the set removed.
This also works for the right side of a string, using the strings.TrimRight
function.
...
s := " Hello, World! "
fmt.Println(len(s)) // 15
s1 := strings.TrimLeft(s, " ")
fmt.Println(s1) // Hello, World!
fmt.Println(len(s1)) // 14
s2 := strings.TrimRight(s, " ")
fmt.Println(s2) // Hello, World!
fmt.Println(len(s2)) // 14
How to Concatenate Strings in Go
To concatenate two strings in Go, you can use the + operator. The + operator can be used to concatenate any number of strings.
...
s1 := "Hello, "
s2 := "World!"
s3 := s1 + s2
fmt.Println(s3) // Hello, World!
We can also use fmt.Sprintf
to concatenate strings. fmt.Sprintf
is a function that returns a string that is the concatenation of the strings passed as arguments. It is similar to the + operator, but it is more flexible because it can be used to concatenate any number of strings.
...
s1 := "Hello, "
s2 := "World!"
s3 := fmt.Sprintf("%s%s", s1, s2)
fmt.Println(s3) // Hello, World!
String concatenation with strings.Builder
The strings.Builder
type is a struct that provides a way to efficiently build a string by writing to it. It is similar to the bytes.Buffer
type, which is used to build a byte slice. The strings.Builder
type is useful when you need to concatenate a large number of strings because it avoids the overhead of creating a new string each time you concatenate two strings thus improving performance.
s1 := "Hello, "
s2 := "World!"
var b strings.Builder
b.WriteString(s1)
b.WriteString(s2)
s3 := b.String()
fmt.Println(s3) // Hello, World!
How to Split Strings in Go
To split a string into a slice of strings, you can use the strings.Split
function. This function takes two arguments: the string to split and the delimiter to split on. It returns a slice of strings that are the result of splitting the string on the delimiter.
...
s := "Hello, World!"
s1 := strings.Split(s, ", ")
fmt.Println(s1) // [Hello World!]
How to Join Strings in Go
To join a slice of strings into a single string, you can use the strings.Join
function. This function takes two arguments: the slice of strings to join and the delimiter to join on. It returns a string that is the result of joining the slice of strings on the delimiter.
...
s := []string{"Hello", "World!"}
s1 := strings.Join(s, ", ")
fmt.Println(s1) // Hello, World!
How to Split Strings by Index in Go
In Go, you can split a string by index using the strings.SplitN()
function. This function takes a string, a separator, and a number of splits as arguments and returns a slice of strings. The following example shows how to split a string by index using the strings.SplitN()
function.
package main
import (
"fmt"
"strings"
)
func main() {
str := "Do or do not, there is no try."
strSlice := strings.SplitN(str, " ", 2)
fmt.Println(strSlice) // [Do or do not, there is no try.]
fmt.Println(len(strSlice)) // 2
}
How to Convert Strings to Numbers in Go
To convert a string to a number in Go, you can use the strconv.Atoi
function or the strconv.ParseInt
function depending on the type of number you want to convert to.
...
s := "123"
i, err := strconv.Atoi(s)
if err != nil {
fmt.Println(err)
}
fmt.Println(i) // 123
...
s := "123"
i, err := strconv.ParseInt(s, 10, 64)
if err != nil {
fmt.Println(err)
}
fmt.Printf("%T\n", i) // int64
How to Check if a String Contains a Substring in Go
To check if a string contains a substring in Go, you can use the strings.Contains
function. This function takes two arguments: the string to check and the substring to check for. It returns a boolean indicating whether the string contains the substring.
...
s := "Hello, World!"
fmt.Println(strings.Contains(s, "Hello")) // true
fmt.Println(strings.Contains(s, "World")) // true
fmt.Println(strings.Contains(s, "Foo")) // false
How to Replace a Substring in a String in Go
To replace a substring in a string in Go, you can use the strings.Replace
function. This function takes four arguments: the string to replace, the substring to replace, the replacement string, and the number of times to replace. It returns a string with the substring replaced.
...
s := "Golang is awesome!"
s1 := strings.Replace(s, "awesome", "great", 1)
fmt.Println(s1) // Golang is great!
You could also use the strings.ReplaceAll
function to replace all occurrences of a substring in a string.
...
s := "Golang is awesome and awesome!"
s1 := strings.ReplaceAll(s, "awesome", "great")
fmt.Println(s1) // Golang is great and great!
How to Reverse a String in Go
To reverse a string in Go, you need to convert the string to a slice of runes. You can then a for loop to iterate over the slice of runes and append each rune to a new slice of runes. Finally, you can convert the slice of runes to a string.
...
s := "Hello, World!"
r := []rune(s)
for i, j := 0, len(r)-1; i < j; i, j = i+1, j-1 {
r[i], r[j] = r[j], r[i]
}
s1 := string(r)
fmt.Println(s1) // !dlroW ,olleH
The problem with this approach is that it requires you to convert the string to a slice of runes and then back to a string. This is not very efficient. A better approach is to use the strings.Builder
type. You can use the strings.Builder
type to build a string by appending it to it. You can then use the strings.Builder.String
method to get the string.
...
s := "Hello, World!"
var b strings.Builder
for i := len(s) - 1; i >= 0; i-- {
b.WriteByte(s[i])
}
s1 := b.String()
fmt.Println(s1) // !dlroW ,olleH
When dealing with encodings you can use the unicode/utf8
package to reverse a string. The unicode/utf8
package provides functions to decode and encode UTF-8 encoded strings. You can use the utf8.DecodeRuneInString
function to decode a single UTF-8 encoded rune from a string. You can then use the utf8.EncodeRune
function to encode a single UTF-8 encoded rune to a string.
...
s := "The quick brown 狐 jumped over the lazy 犬"
var b strings.Builder
for len(s) > 0 {
r, size := utf8.DecodeRuneInString(s)
b.WriteRune(r)
s = s[size:]
}
s1 := b.String()
fmt.Println(s1) // 犬 yzal eht revo depmuj 狐 nworb kciuq ehT
How to Convert a String to Uppercase or Lowercase in Go
To convert a string to uppercase in Go, you can use the strings.ToUpper
function. The function returns a string with all the characters in uppercase.
...
s := "Hello, World!"
s1 := strings.ToUpper(s)
fmt.Println(s1) // HELLO, WORLD!
To convert a string to lowercase in Go, you can use the strings.ToLower
function. The function returns a string with all the characters in lowercase.
...
s := "Hello, World!"
s1 := strings.ToLower(s)
fmt.Println(s1) // hello, world!
How to Convert a String to Title Case in Go
To convert a string to title case in Go, you can use the cases.Title
function from the golang.org/x/text/cases
package.
This function takes a language tag and a string as arguments and returns a string with the first letter of each word in uppercase.
Install the golang.org/x/text/cases
Package
To install the golang.org/x/text/cases
package, you can use the go get
command.
go get golang.org/x/text/cases
go get golang.org/x/text/language
...
import "golang.org/x/text/cases"
import "golang.org/x/text/language"
...
s := "hello, world!"
s1 := cases.Title(language.English).String(s)
fmt.Println(s1) // Hello, World!
strings.Title
was deprecated in Go 1.18 and replaced by the cases.Title
function. Though strings.Title
still works, it is recommended to use the cases.Title
function instead or use the strings.ToTitle
function.
...
str := "dz"
fmt.Println(strings.ToTitle(str)) // Dz
fmt.Println(strings.ToUpper(str)) // Dz
How to Check if a String starts or ends with a Substring in Go
To check if a string starts with a substring in Go, you can use the strings.HasPrefix
function. It returns a boolean indicating whether the string starts with the substring.
...
s := "Hello, World!"
fmt.Println(strings.HasPrefix(s, "Hello")) // true
fmt.Println(strings.HasPrefix(s, "World")) // false
To check if a string ends with a substring in Go, you can use the strings.HasSuffix
function. It returns a boolean indicating whether the string ends with the substring.
...
s := "Hello, World!"
fmt.Println(strings.HasSuffix(s, "Hello")) // false
fmt.Println(strings.HasSuffix(s, "World!")) // true
How to find the Index of a Substring in a String in Go
To find the index of a substring in a string in Go, you can use the strings.Index
function. It returns the index of the first occurrence of the substring in the string.
...
s := "Hello, World!"
fmt.Println(strings.Index(s, "Hello")) // 0
fmt.Println(strings.Index(s, "World!")) // 7
fmt.Println(strings.Index(s, "Foo")) // -1
If the substring is not found in the string, the function returns -1
.
To find the index of the last occurrence of a substring in a string in Go, you can use the strings.LastIndex
function.
...
// I know this doesn't make sense, but it's just an example.
s := "Data is the new oil and oil is the new data"
fmt.Println(strings.LastIndex(s, "data")) // 39
fmt.Println(strings.LastIndex(s, "oil")) // 24
How to Count the Number of Occurrences of a Substring in a String in Go
To count the number of occurrences of a substring in a string in Go, you can use the strings.Count
function. It returns the number of occurrences of the substring in the string.
...
s := "data is the new oil and oil is the new data"
fmt.Println(strings.Count(s, "data")) // 2
fmt.Println(strings.Count(s, "oil")) // 2
How to convert boolean to string in Go
To convert a boolean to a string in Go, you can use the strconv.FormatBool
function. It returns a string representation of the boolean.
...
b := true
s := strconv.FormatBool(b)
fmt.Println(s) // true
fmt.Printf("%T", s) // string
How to compare strings in Go
To compare strings in Go, you can use the ==
operator. It returns a boolean indicating whether the strings are equal.
...
s1 := "Hello, World!"
s2 := "Hello, World!"
fmt.Println(s1 == s2) // true
How to convert a string to a byte slice in Go
To convert a string to a byte slice in Go, you can use the []byte
type conversion. This will return a byte slice that represents the string.
...
s := "Hello, World!"
b := []byte(s)
fmt.Println(b) // [72 101 108 108 111 44 32 87 111 114 108 100 33]
We have done this step before in the section about reversing a string.
Conclusion
String manipulation is a common task in programming. In this article, we have seen how to manipulate strings in Go. We have seen various functions to manipulate strings in Go using the strings
package, cases
package, and strconv
package.