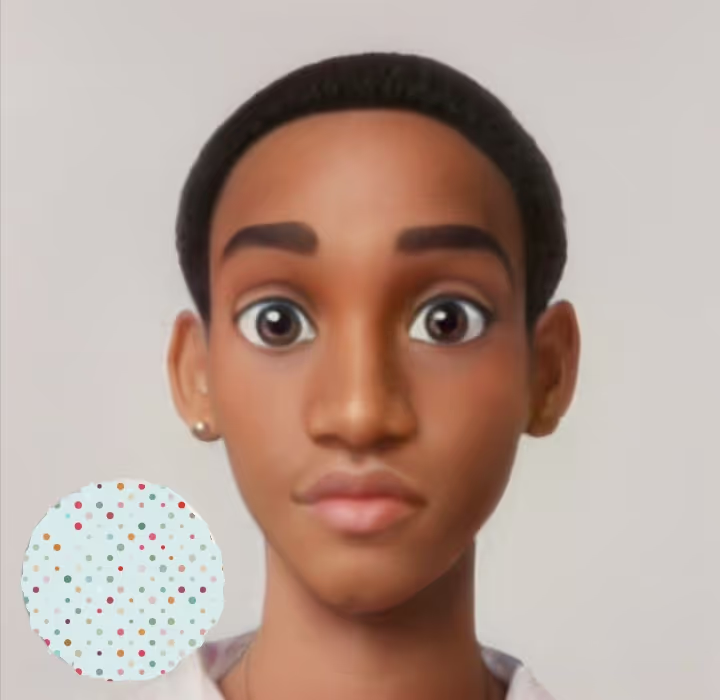
How To Pretty-Print JSON in Go
Introduction
Pretty printing JSON data is a common task when working with JSON in Go. It makes the data more readable and easier to understand. We all know how ugly the cli output can be when we print JSON data without pretty-printing unless we use a tool like jq
to format the output.
Pretty-Print JSON in Go
1. MarshalIndent
To pretty-print JSON data in Go, MarshalIndent
can be used. The function is provided by the encoding/json
package and takes three arguments: the data to be marshaled, a prefix string, and the indentation string. The prefix string is used to indent the first line of the output, and the indentation string is used to indent subsequent lines.
The following example shows how to pretty-print JSON data in Go:
package main
import (
"encoding/json"
"fmt"
)
func main() {
data := map[string]interface{}{
"name": "Kevin",
"age": 30,
"address": map[string]interface{}{
"street": "123 Main St",
"city": "New York",
"state": "NY",
},
}
json, err := json.MarshalIndent(data, "", " ")
if err != nil {
panic(err)
}
fmt.Println(string(json))
}
The output of the above program is:
{
"name": "Kevin",
"age": 30,
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY"
}
}
2. json.Indent
json.Indent
is another way to pretty-print JSON data in Go. The function takes three arguments: a bytes.Buffer
to write the output to, the data to be indented, a prefix string, and the indentation string. The prefix string is used to indent the first line of the output, and the indentation string is used to indent subsequent lines.
The difference between the two methods is that json.Indent
does not return the indented JSON data, but instead writes it to the bytes.Buffer
passed as the first argument. This means that the output of json.Indent
must be printed separately.
package main
import (
"bytes"
"encoding/json"
"fmt"
)
func main() {
data := map[string]interface{}{
"name": "Kevin",
"age": 30,
"address": map[string]interface{}{
"street": "123 Main St",
"city": "New York",
"state": "NY",
},
}
jsonData, err := json.Marshal(data)
if err != nil {
panic(err)
}
var buf bytes.Buffer
err = json.Indent(&buf, jsonData, "", " ")
if err != nil {
panic(err)
}
fmt.Println(buf.String())
}
The output of the above program is:
{
"name": "Kevin",
"age": 30,
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY"
}
}
3. NewEncoder.SetIndent
json.NewEncoder
provides a SetIndent
method that can be used to pretty-print JSON data in Go. The method takes two arguments: a prefix string and the indentation string.
package main
import (
"encoding/json"
"os"
)
func main() {
data := map[string]interface{}{
"name": "Kevin",
"age": 30,
"address": map[string]interface{}{
"street": "123 Main St",
"city": "New York",
"state": "NY",
},
}
encoder := json.NewEncoder(os.Stdout)
encoder.SetIndent("", " ")
if err := encoder.Encode(data); err != nil {
panic(err)
}
}
If you are only printing json data to the console, you can pipe the output
of your program to the jq
command to pretty-print the JSON
data. For example, go run main.go | jq
.
Conclusion
In this article, we explored three ways to pretty-print JSON data in Go: MarshalIndent
, json.Indent
, and NewEncoder.SetIndent
. The first two return indented data, while the last writes it to the buffer. It’s essential to know how to manually pretty-print JSON data, even though libraries exist.