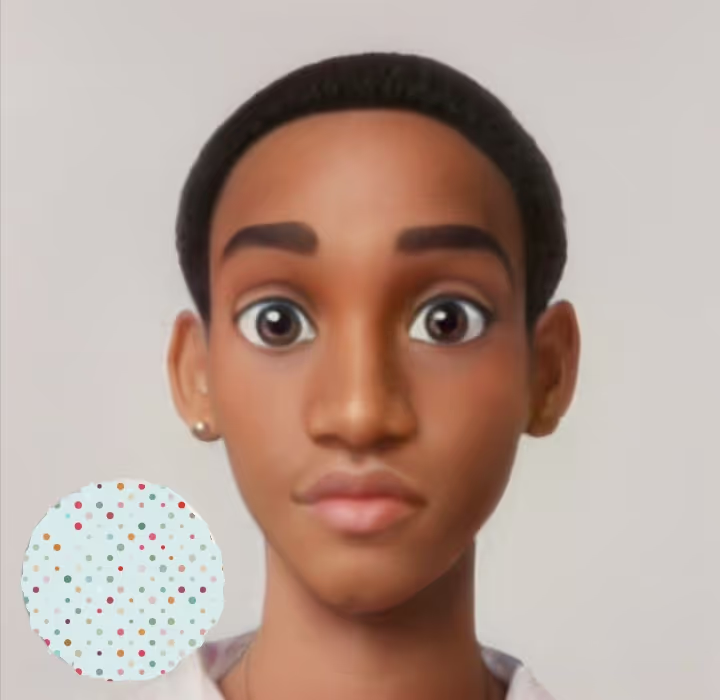
Golang Environment Variables
Introduction
In this article, we will explore the use of environment variables in the Go programming language, commonly referred to as Golang. We will cover the basics of what environment variables are, how they can be used in Golang, and some best practices for utilizing them in your applications. Whether you are a seasoned Go developer or new to the language, this guide will provide valuable insights into working with environment variables in Golang.
What are Environment Variables?
Environment variables are a way to store and retrieve information, such as configuration settings, on a computer system. They are typically stored in the operating system and can be accessed and modified by programs and scripts running on the system. Environment variables can be used to store information such as file paths, server addresses, or credentials. They are often used to separate sensitive information from code and to make it easier to change settings without modifying the code itself.
Environment Variables in Golang
Environment variables in Golang are no different than in any other programming language. In Golang, environment variables can be accessed using the os
package.
How to Get Environment Variables in Golang
To get an environment variable in Golang, you can use the os.Getenv
function. This function takes a string as an argument and returns the value of the environment variable as a string. If the environment variable does not exist, it will return an empty string.
package main
import (
"fmt"
"os"
)
func main() {
api_Key := os.Getenv("API_KEY")
fmt.Println(api_Key)
}
How to get all Environment Variables in Golang
To get all environment variables in Golang, you can use the os.Environ
function. This function returns a key-value pair of all environment variables as a slice of strings. Each string in the slice is formatted as key=value
.
package main
import (
"fmt"
"os"
)
func main() {
envs := os.Environ()
for _, e := range envs {
fmt.Println(e)
}
}
This code will print all the environment variables in the current process along with their values to the console. You can loop through the slice and print each environment variable and its value individually.
How to Set Environment Variables in Golang
To set an environment variable in Golang, you can use the os.Setenv
function. This function takes two strings as arguments, the name of the environment variable and the value to set it to. It returns an error if the environment variable could not be set.
package main
import (
"fmt"
"os"
)
func main() {
os.Setenv("SomeVar", "SomeValue")
someVar := os.Getenv("SomeVar")
fmt.Println(someVar)
}
How to Unset Environment Variables in Golang
To unset an environment variable in Golang, you can use the os.Unsetenv
function. This function takes a string as an argument, the name of the environment variable to unset. It returns an error if the environment variable could not be unset.
package main
import (
"fmt"
"os"
)
func main() {
os.Setenv("SomeVar", "SomeValue")
someVar := os.Getenv("SomeVar")
fmt.Println(someVar)
os.Unsetenv("SomeVar")
someVar = os.Getenv("SomeVar")
fmt.Println(someVar)
}
Get Environment Variables in Golang with godotEnv
The os
package is a great way to get and set environment variables in Golang. However, it can be tedious to set environment variables in the operating system and then access them in your application. It is also not very convenient to set environment variables in the operating system when you are developing your application.
This is where the godotenv
package comes in. The godotenv
package is a go library that loads environment variables from a .env
file. This makes it easy to set environment variables in a file and then access them in your application. It also makes it easy to set environment variables when you are developing your application.
How to Install the godotEnv Package
To install the godotenv
package, you can use the go get
command.
go get github.com/joho/godotenv
How to Use the godotEnv Package
To use the godotenv
package, you can import it into your application.
...
import "github.com/joho/godotenv"
...
To load environment variables from a .env
file, you can use the godotenv.Load
function. This function returns an error if the .env
file could not be loaded.
API_KEY=1234567890
...
err := godotenv.Load()
if err != nil {
log.Fatal("Error loading .env file")
}
...
You can then access the environment variables in your application using the os.Getenv
function.
...
api_Key := os.Getenv("API_KEY")
fmt.Println(api_Key)
...
The output of this code will be 1234567890
.
Best Practices for Using Environment Variables in Golang
Use Consistent Naming Conventions
When naming environment variables, it is best to use consistent naming conventions. This will make it easier to identify and manage environment variables. It is also a good idea to use a prefix for all environment variables to make it easier to identify them. For example, you could use the name of your application as a prefix for all environment variables.
Set default values for environment variables when they are not defined.
When using environment variables in your application, it is a good idea to set default values for them. This will ensure that your application will still run even if the environment variables are not defined.
Be mindful of the scope of the environment variables, changes made to environment variables will only be visible to the process that made the change and its children.
Be careful when using environment variables for sensitive information such as passwords, keys, and secrets. Be sure to encrypt and protect them and use them only in a secure way.
Conclusion
Environment variables are a great way to store and retrieve information on a computer system. They are often used to store sensitive information such as passwords, keys, and secrets. In this article, we learned how to get, set and unset environment variables in Golang. We also learned how to use the godotenv
package to load environment variables from a .env
file. We also learned some best practices for using environment variables in Golang.