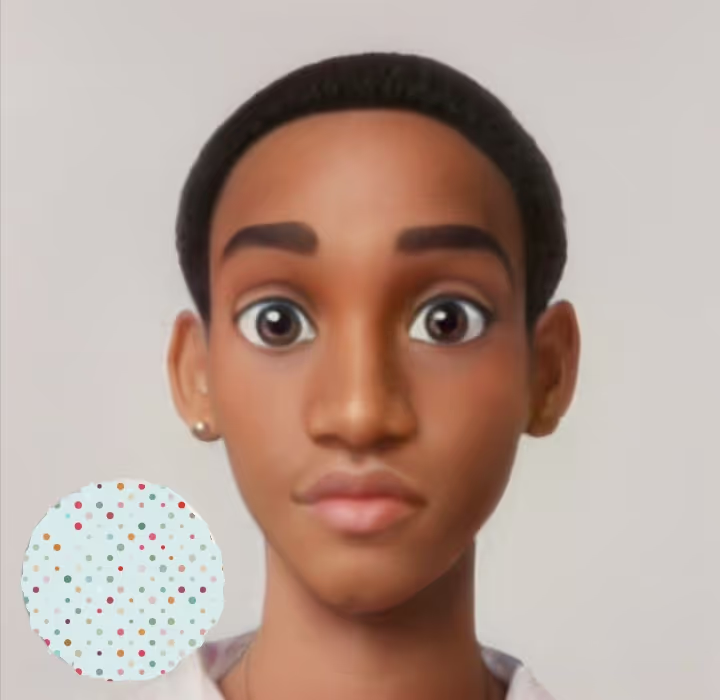
Golang Base64 Encoding and Decoding
Introduction
Base64 is a standard encoding for converting binary data to text format. Base64 is commonly used for encoding binary data in text formats such as JSON, XML, and HTML. Base64 is also used for encoding binary data in email attachments.
Base64 Encoding in Go
Encoding is the process of converting data from one format to another. In this case, we are converting binary data to text. The base64
package provides the EncodeToString
function for encoding binary data to base64.
package main
import (
"encoding/base64"
"fmt"
)
func main(){
data := []byte("Hello World!")
encoded := base64.StdEncoding.EncodeToString(data)
fmt.Println(encoded)
}
The EncodeToString
function takes a byte slice as input and returns a string. The StdEncoding
variable is a *Encoding
type that implements the Encoding
interface. The Encoding
interface provides the EncodeToString
function.
The output of the program is:
SGVsbG8gV29ybGQh
Base64 Decoding in Go
Decoding is the process of converting data from one format to another. In this case, we are converting text to binary data. The base64
package provides the DecodeString
function for decoding base64 to binary data.
package main
import (
"encoding/base64"
"fmt"
)
func main(){
data := []byte("SGVsbG8gV29ybGQh")
decoded, err := base64.StdEncoding.DecodeString(string(data))
if err != nil {
fmt.Println(err)
}
fmt.Println(string(decoded))
}
The DecodeString
function takes a string as input and returns a byte slice. The StdEncoding
variable is a *Encoding
type that implements the Encoding
interface. The Encoding
interface provides the DecodeString
function.
The output of the program is:
Hello World!
Url Encoding and Decoding
The base64
package provides the URLEncoding
variable for encoding and decoding base64 using the URL and filename safe alphabet. The URLEncoding
variable is a *Encoding
type that implements the Encoding
interface. The Encoding
interface provides the EncodeToString
and DecodeString
functions.
package main
import (
"encoding/base64"
"fmt"
)
func main(){
data := []byte("Hello World!")
encoded := base64.URLEncoding.EncodeToString(data)
fmt.Println(encoded)
decoded, err := base64.URLEncoding.DecodeString(encoded)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(decoded))
}
This program functions the same as the previous program but uses the URLEncoding
variable instead of the StdEncoding
variable.
The output of the program is:
SGVsbG8gV29ybGQh
Hello World!
Raw Encoding and Decoding
The base64
package provides the RawStdEncoding
variable for encoding and decoding base64 using the standard raw, unpadded base64 format. The RawStdEncoding
variable is a *Encoding
type that implements the Encoding
interface. The Encoding
interface provides the EncodeToString
and DecodeString
functions.
package main
import (
"encoding/base64"
"fmt"
)
func main(){
data := []byte("Hello World!")
encoded := base64.RawStdEncoding.EncodeToString(data)
fmt.Println(encoded)
decoded, err := base64.RawStdEncoding.DecodeString(encoded)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(decoded))
}
Raw encoding and decoding are useful when encoding binary data that needs to be stored in a text format. The output of the program is:
SGVsbG8gV29ybGQh
Hello World!
Example: Encoding and Decoding a JSON Object
The base64
package provides the EncodeToString
and DecodeString
functions for encoding and decoding base64. The json
package provides the Marshal
and Unmarshal
functions for encoding and decoding JSON. The json
package also provides the MarshalIndent
function for encoding JSON with indentation.
package main
import (
"encoding/base64"
"encoding/json"
"fmt"
)
type Person struct {
Name string
Age int
}
func main(){
p := Person{
Name: "John Doe",
Age: 30,
}
data, err := json.MarshalIndent(p, "", " ")
if err != nil {
fmt.Println(err)
}
encoded := base64.StdEncoding.EncodeToString(data)
fmt.Println(encoded)
decoded, err := base64.StdEncoding.DecodeString(encoded)
if err != nil {
fmt.Println(err)
}
var p2 Person
err = json.Unmarshal(decoded, &p2)
if err != nil {
fmt.Println(err)
}
fmt.Println(p2)
}
The MarshalIndent
function takes a value and returns a byte slice. The MarshalIndent
function also takes a prefix and an indent string. The prefix is prepended to each line of the output. The indent string is used to indent each level of the output. The MarshalIndent
function is useful for encoding JSON with indentation.
The Unmarshal
function takes a byte slice and a pointer to a value. The Unmarshal
function decodes the JSON-encoded data and stores the result in the value pointed to by v. The Unmarshal
function returns an error if the JSON-encoded data is not a valid representation of the value pointed to by v.
The output of the program is:
eyJBY2UiOjMwLCJOYW1lIjoiSm9obiBEb2UifQ==
{30 John Doe}
Other Functions in the Base64 Package
NewEncoder
- returns a new base64 stream encoder. Writes to the returned writer encode base64 data to w.NewDecoder
- returns a new base64 stream decoder. Reads from r decode base64 data to w.NewEncoding
- returns a new custom Encoding defined by the given alphabet, which must be a 64-byte string that does not contain the padding character ’=‘.RawStdEncoding
- returns the standard raw, unpadded base64 encoding.
Other Types in the Base64 Package
Encoding
- represents a base64 encoding/decoding scheme, defined by a 64-character alphabet. The most common usage is via the standard predefined Encodings StdEncoding and URLEncoding.CorruptInputError
- an error that reports that the input is not valid base64 data.
Conclusion
In this article, we looked at how to encode and decode base64 in Go. We looked at the base64
package and the EncodeToString
and DecodeString
functions. We also looked at the URLEncoding
and RawStdEncoding
variables.