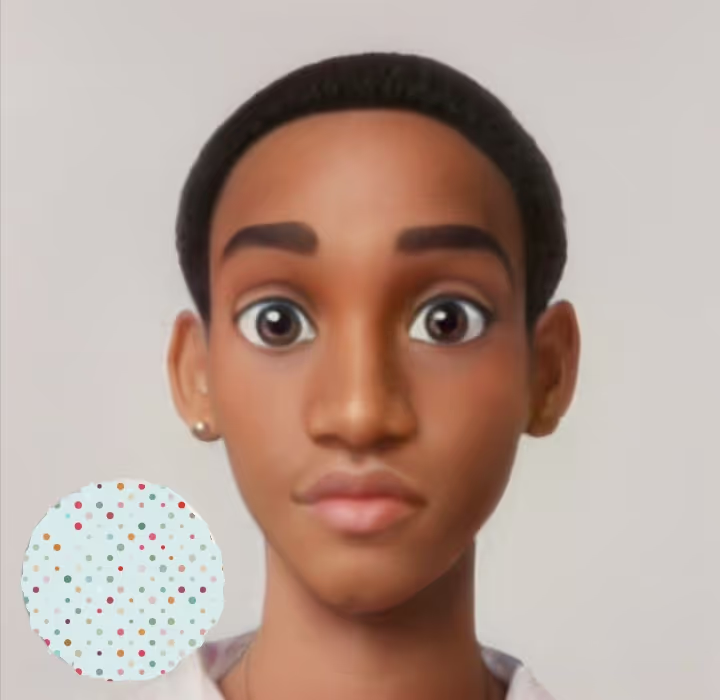
Golang Anonymous Structs
What are Anonymous Structs in Golang?
An anonymous struct is a struct without a name. It is used to create a struct on the fly without having to define a struct type. It is also useful when you want to create a struct with a single field.
How to create an Anonymous Struct in Golang
To create an anonymous struct, you can use the following syntax:
...
<var>:= struct {
<field> type
}{
field: value,
}
...
In the above syntax, <var>
is the variable name of the anonymous struct, <field>
is the field name of the anonymous struct, and type
is the type of the field. The value of the field is assigned to the variable using the field: value
syntax. The value of the field can be any valid value of the type of field.
Example:
...
s1 := struct {
name string
age int
}{
name: "John",
age: 30,
}
...
How to access the fields of an Anonymous Struct in Golang
To access the fields of an anonymous struct the .
operator can be used. The syntax is as follows:
...
s1 := struct {
name string
age int
}{
name: "John",
age: 30,
}
fmt.Println(s1.name) // John
fmt.Println(s1.age) // 30
Anonymous struct array in Golang
Anonymous structs can be used to create an array of structs. The syntax is as follows:
...
s := [3]struct {
name string
age int
}{
{
name: "John",
age: 30,
},
{
name: "Mary",
age: 25,
},
{
name: "Peter",
age: 35,
},
}
fmt.Println(s[0].name) // John
fmt.Println(s[0].age) // 30
fmt.Println(s[1].name) // Mary
fmt.Println(s[1].age) // 25
fmt.Println(s[2].name) // Peter
fmt.Println(s[2].age) // 35
In the example above, an array of anonymous structs is created. The array has 3 elements. Each element is an anonymous struct with the name
and age
fields.
Since slices are built on top of arrays, anonymous structs can also be used to create a slice of structs.
Anonymous struct with a method in Golang
Anonymous structs can also have methods. The syntax is as follows:
...
s1 := struct {
name string
age int
}{
name: "John",
age: 30,
}
func (s struct {
name string
age int
}) greet() {
fmt.Println("Hello", s.name)
}
s1.greet() // Hello John
In the example above, a method greet()
for the anonymous struct s1
is created. The method greet()
takes the anonymous struct as a receiver. The greet()
method can be called on the s1
variable.
Anonymous struct interface in Golang
Anonymous structs can also implement an interface. The syntax is as follows:
...
type person interface {
greet()
}
s1 := struct {
name string
age int
}{
name: "John",
age: 30,
}
func (s struct {
name string
age int
}) greet() {
fmt.Println("Hello", s.name)
}
func sayHello(p person) {
p.greet()
}
sayHello(s1) // Hello John
In the example above, the anonymous struct s1
implements the person
interface. The sayHello()
function takes the person
interface as a parameter. The s1
variable is passed to the sayHello()
function. The s1
variable implements the person
interface, so it can be passed to the sayHello()
function.
Nested Anonymous Structs in Golang
Anonymous structs can be nested. The syntax is as follows:
...
s1 := struct {
name string
age int
address struct {
street string
city string
}
}{
name: "John",
age: 30,
address: struct {
street string
city string
}{
street: "Main Street",
city: "New York",
},
}
fmt.Println(s1.name) // John
fmt.Println(s1.age) // 30
fmt.Println(s1.address.street) // Main Street
fmt.Println(s1.address.city) // New York
In the example above, the address
field of the anonymous struct s1
is a nested anonymous struct.
Conclusion
Anonymous structs are useful when you need to create a struct quickly without defining a struct type. They can also be used to construct an array or a struct slice. Anonymous structs can also implement interfaces and have methods. Anonymous structs can be nested as well.