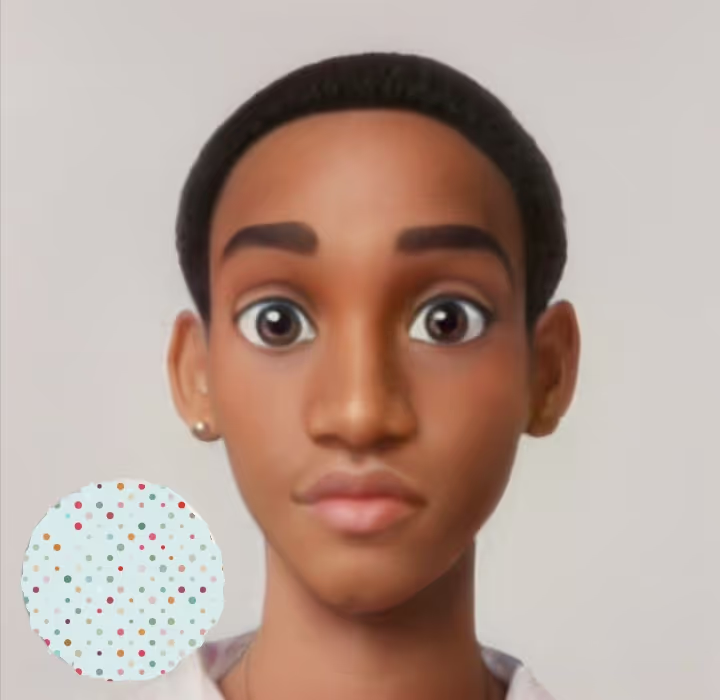
Golang Absolute Value
What is the Absolute Value of a Number?
The absolute value of a number is the distance between the number and zero on a number line. For example, the absolute value of -5 is 5, and that of 5 is 5.
How to Get the Absolute Value of a Number in Golang
To find the absolute value of a number in Golang, use the math.Abs()
function. This function takes a float64
as input and returns a float64
as output.
You have to import the math
package to use this function. You can do this by adding the following line to the top of your file:
...
import "math"
Absolute Value of a Number in Golang Example
Let’s look at an example of how to get the absolute value of a number in Golang.
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println(math.Abs(-5)) // 5
fmt.Println(math.Abs(5)) // 5
}
math.Abs()
Special Cases
If the number is NaN
, math.Abs()
will return NaN
.
If the number is +Inf
, math.Abs()
will return +Inf
.
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println(math.Abs(math.NaN())) // NaN
fmt.Println(math.Abs(math.Inf(1))) // +Inf
}
Absolute Value for int64 in Golang
If you want to get the absolute value of an int64
, you can convert it to a float64
and then use the math.Abs()
function.
package main
import (
"fmt"
"math"
)
func main() {
var num int64 = -5
fmt.Println(math.Abs(float64(num))) // 5
}
You can also use the math.Abs()
function on an int
or int32
by converting it to a float64
.
Application of Absolute Value in Golang
The absolute value of a number is useful for finding the distance between two points on a number line. For example, if you have two points on a number line, you can find the distance between them by subtracting the smaller point from the larger point and then taking the absolute value of the result.
package main
import (
"fmt"
"math"
)
func main() {
var point1 int = 5
var point2 int = 10
var distance int
if point1 > point2 {
distance = point1 - point2
} else {
distance = point2 - point1
}
fmt.Println(math.Abs(float64(distance))) // 5
}