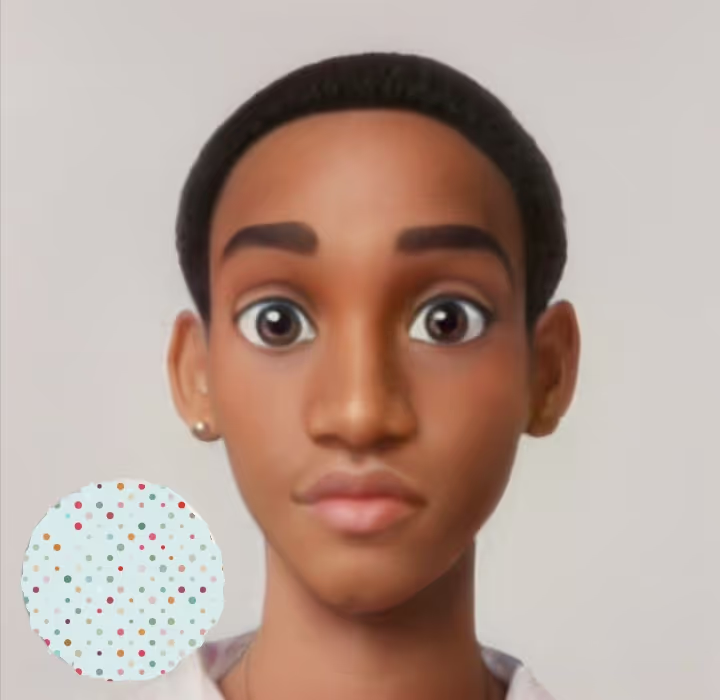
Golang Filepath - (A Complete Guide)
Introduction
The Go programming language has a comprehensive standard library for working with files and directories. The path/filepath
package provides utilities for handling file paths. Filepaths are an essential part of any application that needs to work with files and directories in the filesystem. The path/filepath
package provides several useful functions for working with file paths. This article will provide a comprehensive guide to working with file paths in Go. Let’s get started.
Filepaths in Golang
In Golang, a file path is a string that represents the location of a file or a folder in the file system. Filepaths are used to access and manipulate files and folders programmatically. A file path can be absolute or relative. An absolute file path is a file path that starts with the root folder and provides a complete path to the files or folder(/home/user/file.txt
), on the other hand, a relative path is based on the current folder and provides a path just relative to it(./file.txt
). The path/filepath
package provides several functions for working with file paths in Go.
Working with Filepaths
To work with file paths you’ll need to import the path/filepath
package.
...
import "path/filepath"
Joining Filepaths
To join two or more file paths, the filepath.Join()
function is used. This is a variadic function that takes in several file paths and returns a single file path. This function is useful for constructing file paths from several parts.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.Join("home", "user", "file.txt"))
}
home/user/file.txt
Get Directory of a File or Folder
To get the directory of a file, the filepath.Dir()
function is used. This function takes in a file path and returns the directory of the file.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.Dir("home/user/file.txt"))
}
home/user
Get the Base of a File or Folder
The filepath.Base()
function is used to get the base of a file or folder. This function takes in a file path and returns the base of the file or folder. The base of a file or folder is the last part of the file path.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.Base("home/user/file.txt"))
}
file.txt
This is the inverse of the filepath.Dir()
function.
Get the Absolute Filepath
To get the absolute file path of a file or folder, the filepath.Abs()
function is used. This function takes in a file path and returns the absolute file path of the file or folder. If the file path given is not absolute, it will append the current folder to the file path.
Two values are returned by the filepath.Abs()
function. The first value is the absolute file path and the second value is an error.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.Abs("file.txt"))
}
/home/user/file.txt <nil>
We can also check if a file path is absolute or not using the filepath.IsAbs()
function. This function takes in a file path and returns a boolean value indicating if the file path is absolute or not.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.IsAbs("file.txt"))
}
false
Get the Relative Filepath
The filepath.Rel()
function is used to get the relative file path of a file or folder. This function takes in two file paths, the base path, and the target path, and returns the relative file path of the target path from the base path.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.Rel("home/user", "home/user/file.txt"))
}
file.txt <nil>
Get Matching Files
To get a list of files that match a pattern, the filepath.Glob()
function is used. This function takes in a pattern and returns a list of files that match the pattern. The pattern can be a simple string or a regular expression. If there are no files that match the pattern, an empty list is returned.
package main
import (
"fmt"
"path/filepath"
)
func main() {
files, _ := filepath.Glob("*.txt")
fmt.Println(files)
}
[file.txt]
Get the Extension of a File
To get the extension of a file, the filepath.Ext()
function is used. This function takes in a file path and returns the extension of the file. The extension of a file is the part of the file path after the last .
.
package main
import (
"fmt"
"path/filepath"
)
func main() {
fmt.Println(filepath.Ext("file.txt"))
}
.txt
Conclusion
In this article, we have covered the path/filepath
package. We have seen how to join file paths, and get the directory, among other things. We have covered the most common functions used to work with file paths. For a complete list of functions, check out the path/filepath
package documentation.